
(Doing '\n'.join(…) would also avoid printing two newlines at the end of the output, which is wrong in my opinion.) For this application, you should use '\n'.join(…). Appending just a single character using += involves allocating and copying the entire string.
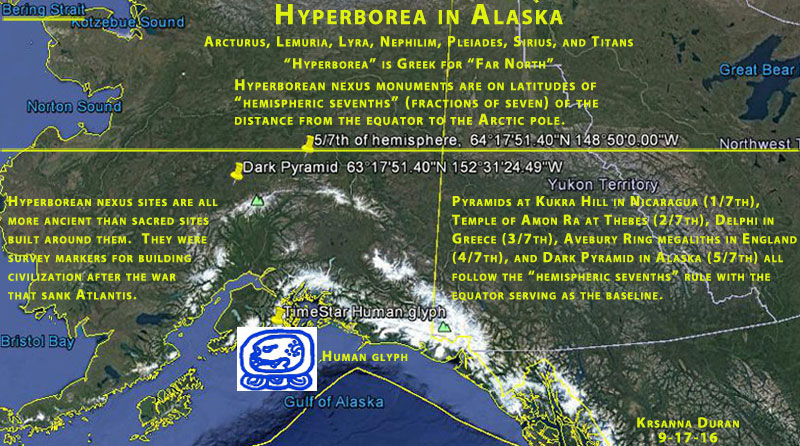
from itertools import chain, cycle, isliceĭef tesselated_triangles(height, cols, rows):ĭef triangle_row(height, cols, space=' ', fill='*'):ĭef alt_triangle_row(height, cols, space=' ', fill='*'): In particular, it is helpful to observe that the odd rows are like the even rows, but upside-down and colour-inverted. The output routine, in turn, can be decomposed into smaller reusable units.
Triangle tessellation validation code#
The input validation code is unrelated to the output routine. It would definitely be a good idea to split the work into functions. In Python, excessive looping can often be remedied using list comprehensions and itertools. Using the * operator to repeat strings is smart, but there are still a lot of nested ifs and and fors. Your code had myself lost over all the top-level variables and conditions leaving me without understanding how each step was correlated to the overall algorithm.Īlso the alternate function is now written and documented by a test, and it may prove useful in other similar ASCII art challenges allowing code re-use. I suggest this method because it is logical and conceptually readable from looking at the code. Using this code to make the odd lines is also easy.Ĭoncatenate a required number of even and odd lines to build the complete output. Return '\n'.join(alternate(' ', '*', ' ', start_space_length, space_length, asterisk_length, cols)įor (start_space_length, asterisk_length, space_length) We can deduce that simply by doing simple cases by hand and counting, or if we are lucky by looking at the output required or given by an equivalent program: def make_triangles(height, cols, rows): Using this function is now easy, we just need to understand how asterisks, spaces, and starting spaces change. Return start * start_length + ''.join(thing * lengthįor thing, length in islice(cycle( ((b,b_length), (a,a_length))), times)) def alternate(a, b, start, start_length, a_length, b_length, times): This action of alternateing spaces and asterisks looks like a fundamental action "building block" of this program so it should be written in its own function. I see the problem as deciding how many asterisks and spaces should go on each line and in which order. I decided to approach the problem bottom-up. Here is some sample output: input: height: 6 cols: 6 rows: 4

Triangle += " " * (half_space + 1) + "*" * stars + " " * half_space Print("Triangles not gonna fit on page, please enter smaller params.
Triangle tessellation full#
Print("make sure shell in full screen so the triangles are viewed properly. The first line is just the normal triangle, the second line and every other line after however only should show half of the triangle on the first and last column. This program has taken me some time to put together and I'm not sure if this is the best way to do this.

It takes the height to form the size of the triangle, cols to specify how many triangles on a line, and rows which is how many lines it outputs. I have this Python project to draw tessellated triangles on the screen.
